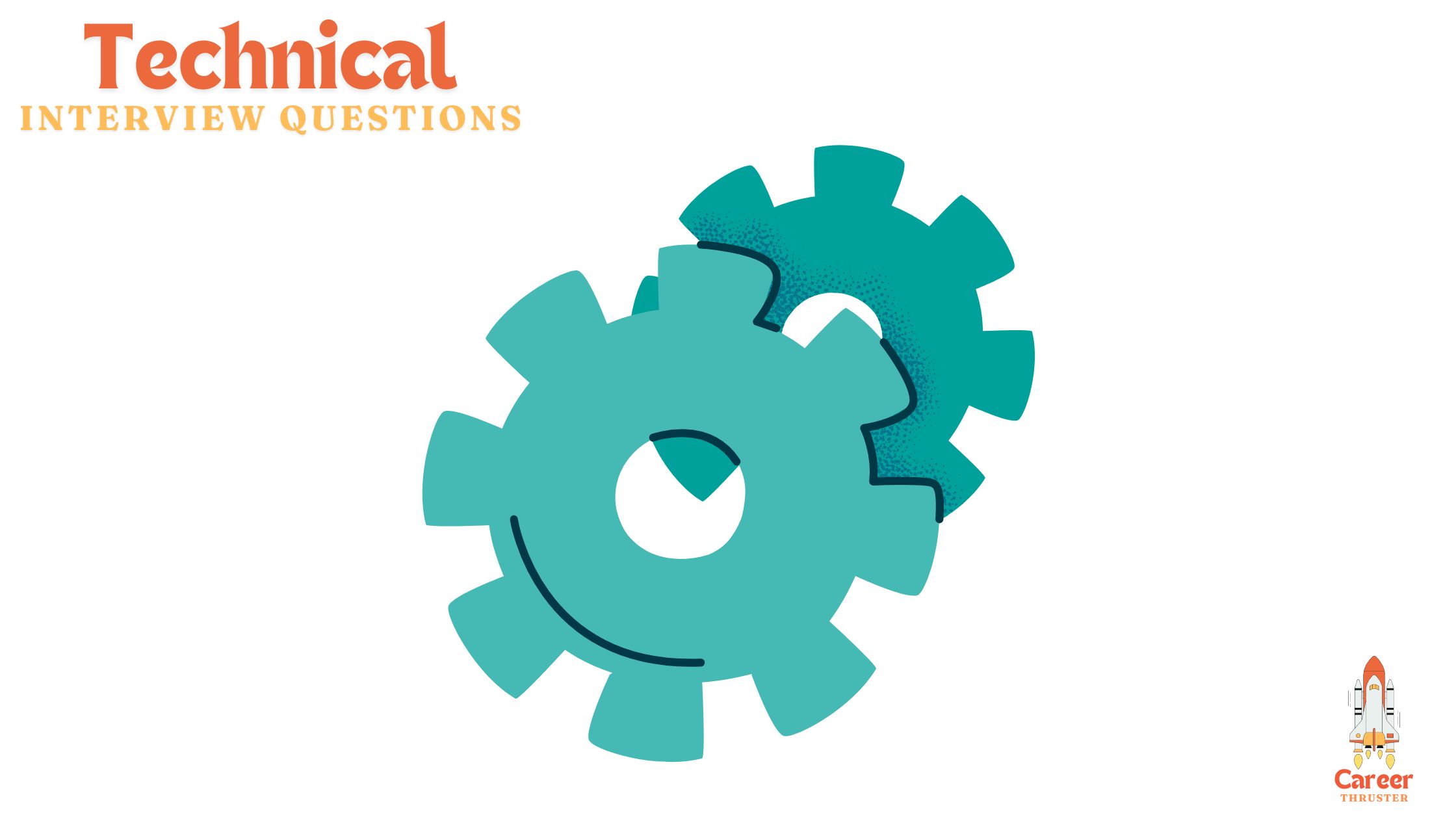
Preparing for a technical interview can feel like standing at the edge of a daunting cliff. You know that what’s ahead could shape your career, and it’s easy to get overwhelmed by the sheer amount of information out there. But you’re not alone in feeling this way. Almost every candidate, from fresh graduates to seasoned professionals, has been in your shoes, wondering what technical interview questions might come their way.
The reality is, technical interviews are designed to test not just what you know, but how you think. They’re a peek into your problem-solving abilities, your approach to coding, and your understanding of core concepts. Instead of seeing them as a series of traps, think of them as an opportunity to showcase your skills and stand out from the crowd. With the right preparation and mindset, you can turn this challenge into a chance to impress your future employers.
In the sections that follow, you’ll find a collection of technical interview questions you might encounter, along with detailed sample answers and tips to help you navigate these questions with confidence. Whether you’re a fresher or someone brushing up on your skills, this guide aims to make your preparation process a bit easier.
Table of Contents
Technical Interview Questions
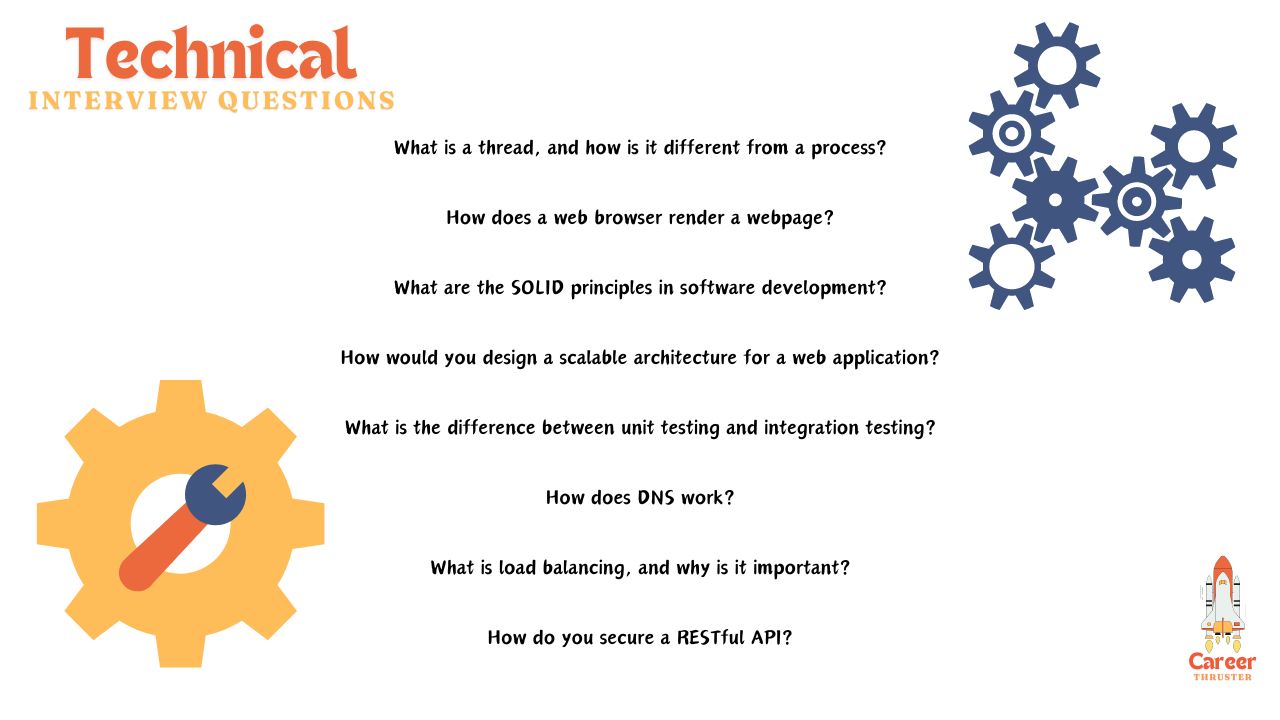
What is the difference between a stack and a queue?
How does a hash table work?
Explain the concept of inheritance in object-oriented programming.
What is the significance of the “static” keyword in Java?
How would you optimize a slow SQL query?
What are the four pillars of object-oriented programming?
Explain the differences between HTTP and HTTPS.
What is recursion, and can you give an example?
How does garbage collection work in Java?
What is a deadlock in multithreading, and how can it be prevented?
What is the difference between GET and POST methods in HTTP?
How does the OS handle memory management?
What is a RESTful API, and how does it differ from SOAP?
Explain polymorphism in the context of OOP.
How would you handle error logging in a large-scale application?
What is the difference between a compiled and an interpreted language?
How does version control work, and why is it important?
What is a race condition, and how can it be avoided?
How would you implement a binary search algorithm?
What are microservices, and what are their advantages?
Explain the concept of normalization in databases.
What is a thread, and how is it different from a process?
How does a web browser render a webpage?
What are the SOLID principles in software development?
How would you design a scalable architecture for a web application?
What is the difference between unit testing and integration testing?
How does DNS work?
What is load balancing, and why is it important?
How do you secure a RESTful API?
Explain the concept of continuous integration and continuous deployment (CI/CD).
Also Read- Team Player Interview Questions
Sample Answers For Technical Interview Questions
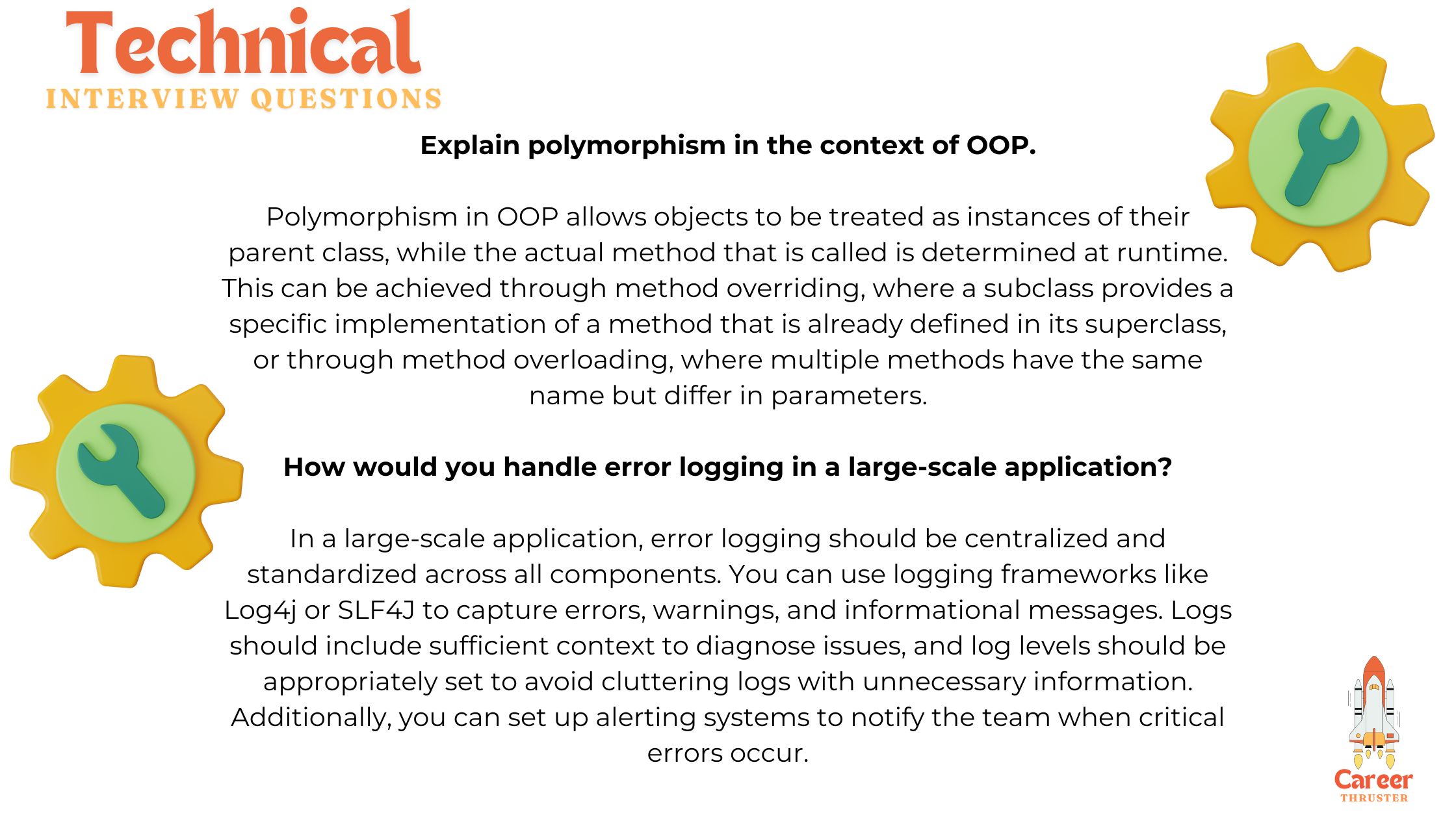
What is the difference between a stack and a queue?
A stack is a data structure that follows the Last In, First Out (LIFO) principle, meaning the last element added is the first to be removed. In contrast, a queue follows the First In, First Out (FIFO) principle, where the first element added is the first to be removed. Stacks are typically used for tasks like function calls, while queues are used in scenarios like task scheduling.
How does a hash table work?
A hash table stores data in key-value pairs, using a hash function to compute an index into an array of buckets, from which the desired value can be found. The efficiency of a hash table comes from its average-case time complexity of O(1) for search, insert, and delete operations, although in the worst case, due to collisions, the time complexity can degrade to O(n).
Explain the concept of inheritance in object-oriented programming.
Inheritance is a mechanism in OOP that allows a new class (called a child or subclass) to inherit properties and methods from an existing class (called a parent or superclass). This promotes code reusability and establishes a relationship between the child and parent classes, where the child can extend or override the inherited features.
What is the significance of the “static” keyword in Java?
The static keyword in Java is used to indicate that a particular variable, method, or block belongs to the class rather than instances of the class. This means that a static member is shared among all instances of the class. It is often used for utility methods or shared resources.
How would you optimize a slow SQL query?
To optimize a slow SQL query, you can start by analyzing the query execution plan to identify bottlenecks. Indexing the columns used in WHERE clauses, avoiding SELECT *, optimizing JOINs, and limiting the use of subqueries can also improve performance. Additionally, caching frequently accessed data and considering database normalization or denormalization depending on the scenario may help.
What are the four pillars of object-oriented programming?
The four pillars of OOP are Encapsulation, Abstraction, Inheritance, and Polymorphism. Encapsulation involves bundling the data and methods that operate on the data within a single unit. Abstraction hides the implementation details and exposes only the necessary aspects. Inheritance allows classes to derive properties and behaviors from other classes. Polymorphism enables a single entity to take multiple forms, typically through method overriding or overloading.
Explain the differences between HTTP and HTTPS.
HTTP (HyperText Transfer Protocol) is a protocol used for transmitting data over the web. HTTPS (HTTP Secure) is the secure version of HTTP, which uses SSL/TLS to encrypt data between the client and server, ensuring data integrity and privacy. HTTPS is essential for protecting sensitive information, like login credentials and payment details.
What is recursion, and can you give an example?
Recursion is a programming technique where a function calls itself to solve smaller instances of a problem until it reaches a base case. An example is the calculation of the factorial of a number. The factorial function (n!) can be defined recursively as n * (n-1)!, with the base case being 0! = 1.
How does garbage collection work in Java?
Garbage collection in Java is an automatic process that frees up memory by identifying and disposing of objects that are no longer in use. The Java Virtual Machine (JVM) periodically performs garbage collection to reclaim memory, using algorithms like Mark and Sweep, where objects are marked as reachable or unreachable, and unreachable objects are removed.
What is a deadlock in multithreading, and how can it be prevented?
A deadlock occurs when two or more threads are blocked forever, waiting for each other to release resources. It can be prevented by avoiding circular wait conditions, using timeout mechanisms, and employing techniques like lock ordering, where locks are always acquired in a specific order to prevent circular dependencies.
What is the difference between GET and POST methods in HTTP?
The GET method is used to request data from a server and can be cached, bookmarked, and remain in the browser history, but it is less secure because data is appended to the URL. The POST method, on the other hand, is used to send data to the server for processing and is more secure since data is not visible in the URL.
How does the OS handle memory management?
The operating system handles memory management through processes like allocation and deallocation of memory space, virtual memory management, paging, and segmentation. It ensures that each process has enough memory to execute while optimizing the use of available memory and preventing memory leaks or corruption.
What is a RESTful API, and how does it differ from SOAP?
A RESTful API is an application programming interface that adheres to REST (Representational State Transfer) principles, using standard HTTP methods for communication and typically returning data in formats like JSON or XML. SOAP (Simple Object Access Protocol), on the other hand, is a protocol that uses XML messaging for communication and includes built-in error handling and security features. REST is often preferred for its simplicity and scalability.
Explain polymorphism in the context of OOP.
Polymorphism in OOP allows objects to be treated as instances of their parent class, while the actual method that is called is determined at runtime. This can be achieved through method overriding, where a subclass provides a specific implementation of a method that is already defined in its superclass, or through method overloading, where multiple methods have the same name but differ in parameters.
How would you handle error logging in a large-scale application?
In a large-scale application, error logging should be centralized and standardized across all components. You can use logging frameworks like Log4j or SLF4J to capture errors, warnings, and informational messages. Logs should include sufficient context to diagnose issues, and log levels should be appropriately set to avoid cluttering logs with unnecessary information. Additionally, you can set up alerting systems to notify the team when critical errors occur.
What is the difference between a compiled and an interpreted language?
A compiled language is transformed into machine code that can be directly executed by the computer’s CPU. Examples include C and C++. An interpreted language, like Python or JavaScript, is executed line by line by an interpreter at runtime, which can be slower than executing compiled code but allows for more flexibility during development.
How does version control work, and why is it important?
Version control systems, like Git, track changes to files over time, allowing multiple people to collaborate on a project while keeping a history of all changes. It is important because it enables teams to work on different parts of a project simultaneously, merge changes, revert to previous versions if necessary, and maintain a clear audit trail of who made what changes and when.
What is a race condition, and how can it be avoided?
A race condition occurs when the outcome of a program depends on the sequence or timing of uncontrollable events, like the order in which threads execute. It can be avoided by using synchronization techniques, such as locks, semaphores, or atomic variables, to ensure that critical sections of code are executed by only one thread at a time.
How would you implement a binary search algorithm?
A binary search algorithm efficiently finds a target value within a sorted array by repeatedly dividing the search interval in half. If the target value is less than the middle element, the search continues in the left half; if greater, in the right half. This process is repeated until the target is found or the interval is empty. The time complexity of binary search is O(log n).
What are microservices, and what are their advantages?
Microservices are an architectural style where a large application is divided into small, independent services, each responsible for a specific functionality. Advantages include improved scalability, easier maintenance, and faster deployment. Microservices also allow teams to work on different services simultaneously, using different technologies if needed, and provide greater fault tolerance since individual services can fail without bringing down the entire system.
Explain the concept of normalization in databases.
Normalization is the process of organizing a database to reduce redundancy and improve data integrity. It involves dividing a database into tables and defining relationships between them according to specific rules, such as removing duplicate data and ensuring that each table contains only related data. The goal is to minimize data anomalies and make the database more efficient to query and update.
What is a thread, and how is it different from a process?
A thread is a lightweight unit of execution within a process, allowing multiple tasks to be performed concurrently within the same program. A process is an independent program in execution, with its own memory space. Threads within the same process share memory, making communication between them faster, but also requiring careful synchronization to avoid issues like race conditions.
How does a web browser render a webpage?
When a web browser renders a webpage, it first fetches the HTML document from the server. The browser then parses the HTML to construct a Document Object Model (DOM) tree, followed by fetching and applying CSS to style the content. Next, it executes any JavaScript to handle dynamic content. Finally, the browser combines the styled DOM tree with other resources like images and fonts to display the page to the user.
What are the SOLID principles in software development?
The SOLID principles are a set of five design principles aimed at making software more maintainable and scalable. They include:
- Single Responsibility Principle (SRP): A class should have only one reason to change, meaning it should have only one job.
- Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.
- Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules; both should depend on abstractions.
How would you design a scalable architecture for a web application?
To design a scalable architecture, you can start by using load balancers to distribute traffic across multiple servers. Implementing a microservices architecture allows scaling individual components independently. Use caching mechanisms like Redis to reduce database load and CDNs to deliver content faster. Employ database replication and sharding to handle increased data loads, and use asynchronous processing to manage high traffic efficiently.
What is the difference between unit testing and integration testing?
Unit testing involves testing individual components or functions of a program in isolation to ensure they work as expected. Integration testing, on the other hand, tests how different components of a system work together. While unit tests are usually faster and easier to write, integration tests provide a broader view of the system’s functionality and how its parts interact.
How does DNS work?
DNS (Domain Name System) translates human-readable domain names into IP addresses that computers use to identify each other on the network. When you enter a domain name in your browser, the DNS server is queried to find the corresponding IP address. This process involves multiple steps, including checking local cache, querying recursive DNS servers, and contacting authoritative DNS servers until the correct IP address is found and returned to the client.
What is load balancing, and why is it important?
Load balancing is the process of distributing network or application traffic across multiple servers to ensure no single server becomes overwhelmed. It is important because it helps maintain high availability, improves performance by preventing bottlenecks, and enhances fault tolerance by rerouting traffic if a server fails. Load balancers can also optimize resource use and provide a seamless user experience even under heavy load.
How do you secure a RESTful API?
Securing a RESTful API involves implementing authentication and authorization mechanisms, such as OAuth2, to control access to the API. You should also use HTTPS to encrypt data in transit, validate and sanitize user inputs to prevent injection attacks, and limit the rate of API requests to prevent abuse. Additionally, regularly update and patch the API to address vulnerabilities and use logging and monitoring to detect and respond to security incidents.
Explain the concept of continuous integration and continuous deployment (CI/CD).
Continuous Integration (CI) involves automatically integrating code changes from multiple contributors into a shared repository several times a day. Automated tests are run to catch errors early. Continuous Deployment (CD) goes a step further by automatically deploying the integrated and tested code to production. CI/CD helps ensure that code is always in a deployable state, reduces the risk of errors, and allows for faster and more reliable releases.
Tips to Answer the Technical Interview Questions
Understand the Question Before You Answer
You can take a moment to fully grasp what the interviewer is asking before you begin to answer. Clarifying the question if needed shows that you’re thoughtful and ensures that your response is relevant. Understanding the context can also help you tailor your answer to the specific requirements of the role.
Break Down Complex Problems
When faced with a complex question, you should break it down into smaller, more manageable parts. Explain your thought process step by step, so the interviewer can follow your logic. This not only helps in delivering a clear answer but also demonstrates your problem-solving approach and communication skills.
Draw From Real Experience
Whenever possible, you can relate your answers to real-life projects or scenarios you’ve encountered. This makes your answers more concrete and credible. If you’re a fresher, discussing your academic projects, internships, or any coding challenges you’ve taken on can show your practical understanding of the concepts.
Practice Coding Out Loud
For coding questions, you should practice writing code on a whiteboard or paper and explaining it out loud. This prepares you to think and communicate simultaneously, which is crucial during technical interviews. Explaining your code as you write it shows your reasoning process and can help the interviewer see how you approach problems.
Keep Learning and Updating Your Knowledge
Technical fields evolve rapidly, so you can stay updated on the latest technologies, tools, and best practices. Demonstrating that you’re up-to-date with industry trends can set you apart from other candidates. Even if a question touches on something you’re not familiar with, showing a willingness to learn can leave a positive impression.
Word Of Advice
Navigating technical interviews might seem challenging, but with the right preparation, you can turn them into opportunities to shine. Remember, these interviews aren’t just about testing your knowledge—they’re about understanding how you think and solve problems.
The questions you’ll face are designed to gauge your technical abilities, but they also reveal your approach to learning and adapting to new challenges. By practicing the questions and sample answers provided, and keeping the tips in mind, you’ll build the confidence needed to handle the toughest questions with ease.
Keep in mind that every interview is a learning experience, whether you succeed or not. Stay curious, keep practicing, and trust in your abilities. With perseverance, you’ll be well on your way to acing your technical interviews and taking the next step in your career.
Pingback: 55+ Team Player Interview Questions (With Sample Answers)