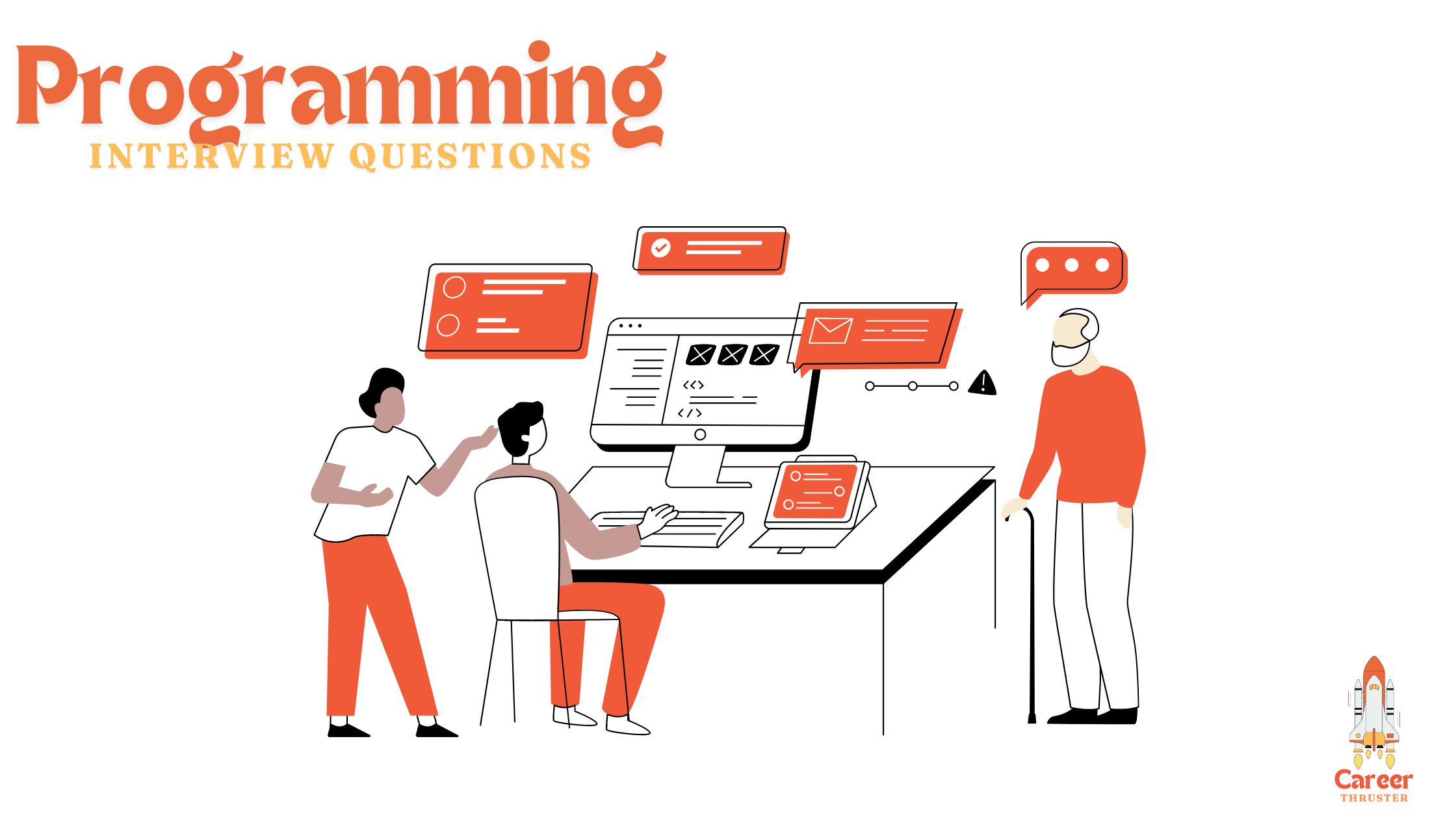
Facing a programming interview can be nerve-wracking, especially when you’re unsure of what questions might come your way. Whether you’re a fresh graduate or an experienced developer, the challenge of answering programming interview questions often feels like a test of not just your technical skills but your problem-solving abilities and composure under pressure.
You might be wondering, “What kind of questions will they ask?” and “How can I best prepare?” Programming interviews are designed to dig deep into your understanding of key concepts, your ability to think on your feet, and how well you can communicate your thought process.
It’s not just about knowing how to code, but also about demonstrating how you approach problems and how adaptable you are in unfamiliar situations. By anticipating common questions and practicing thoughtful, clear responses, you can build the confidence needed to navigate these interviews successfully.
Remember, interviewers are not just looking for the right answer—they want to see how you think, how you handle challenges, and whether you can articulate your ideas effectively.
Table of Contents
Programming Interview Questions
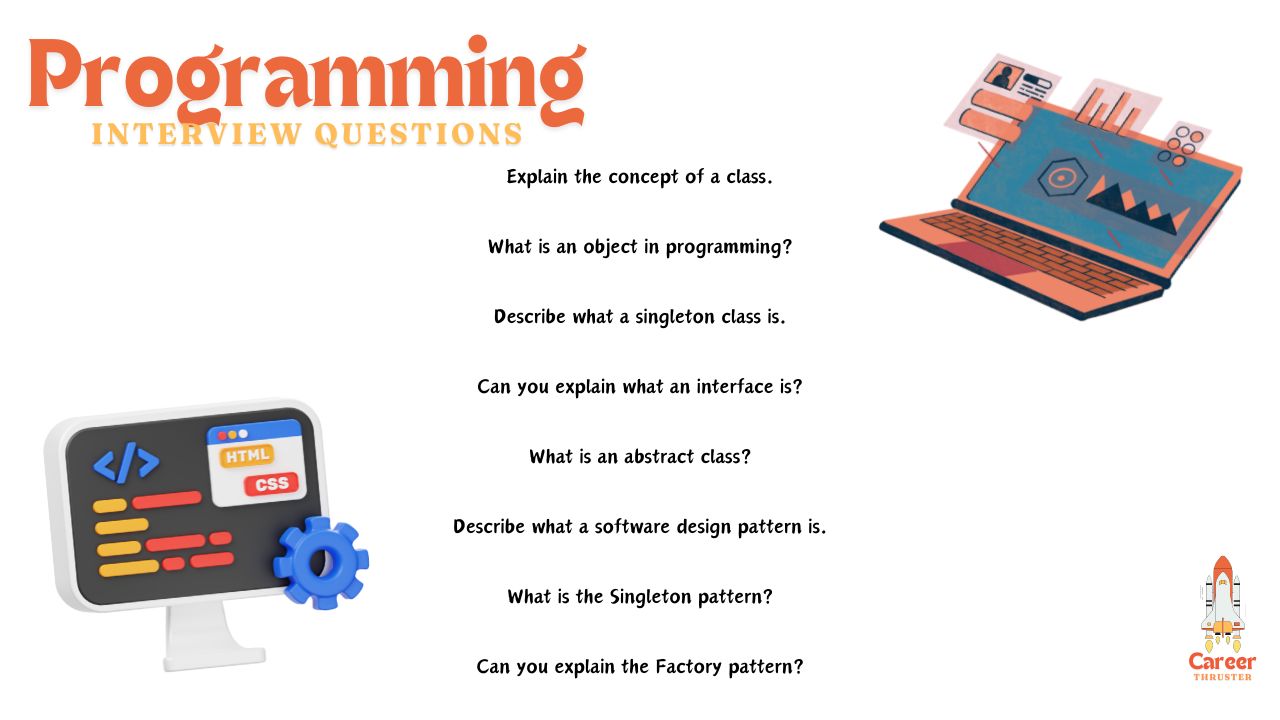
What is a programming language?
Explain the concept of object-oriented programming.
What is a data structure?
Can you explain what a linked list is?
Describe the difference between an array and a linked list.
What is a stack and how does it function?
Explain what a queue is and its primary operations.
What is a binary tree?
Describe what a hash table is.
Can you explain recursion?
What is a pointer and how is it used?
Explain the concept of a null pointer.
What are the differences between stack and heap memory?
What is dynamic programming?
Can you explain what a compiler does?
Describe what an interpreter is.
What is the difference between a compiler and an interpreter?
Explain the concept of garbage collection.
What is a memory leak?
Can you explain what a segmentation fault is?
What are the four principles of OOP (Object-Oriented Programming)?
Describe what inheritance is in OOP.
Can you explain polymorphism?
What is encapsulation in OOP?
Describe abstraction in OOP.
What is a constructor in programming?
Explain what a destructor is.
What is a virtual function?
Can you describe method overloading?
What is method overriding?
Explain the concept of a class.
What is an object in programming?
Describe what a singleton class is.
Can you explain what an interface is?
What is an abstract class?
Describe what a software design pattern is.
What is the Singleton pattern?
Can you explain the Factory pattern?
What is the Observer pattern?
Describe the Model-View-Controller (MVC) pattern.
What is the difference between a process and a thread?
Explain multithreading.
What is a deadlock in programming?
Can you describe what a race condition is?
What is a semaphore?
Explain what a mutex is.
What is a critical section?
Describe what a lambda function is.
What is the difference between synchronous and asynchronous programming?
Explain what RESTful web services are.
Also Read- Problem Solving Interview Questions
Sample Answers For Programming Interview Questions
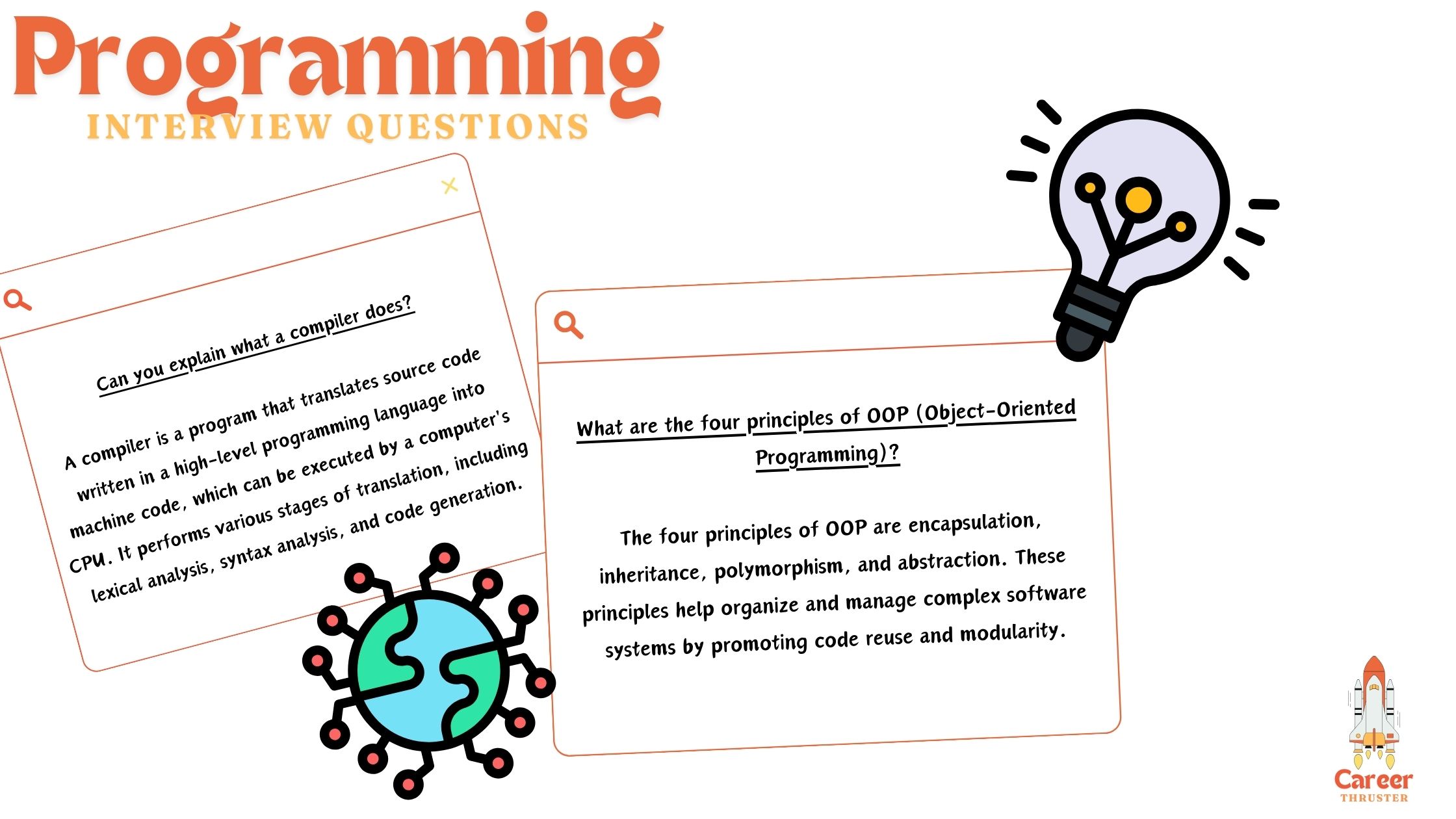
What is a programming language?
A programming language is a formal set of instructions that can be used to produce various kinds of output. These languages are used in computer programming to implement algorithms. Examples include Python, Java, C++, and JavaScript.
Explain the concept of object-oriented programming.
Object-oriented programming (OOP) is a programming paradigm that uses objects and classes to create models based on the real world. It focuses on the concepts of objects that have properties and behaviors, and it emphasizes reusability, encapsulation, inheritance, and polymorphism.
What is a data structure?
A data structure is a way of organizing and storing data in a computer so that it can be accessed and modified efficiently. Common data structures include arrays, linked lists, stacks, queues, trees, and hash tables.
Can you explain what a linked list is?
A linked list is a linear data structure where each element (node) contains a data part and a reference (link) to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory locations.
Describe the difference between an array and a linked list.
An array is a collection of elements stored at contiguous memory locations, whereas a linked list consists of elements (nodes) that are linked using pointers. Arrays allow random access to elements, while linked lists support efficient insertion and deletion.
What is a stack and how does it function?
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. Elements can be added (pushed) and removed (popped) only from the top of the stack.
Explain what a queue is and its primary operations.
A queue is a linear data structure that follows the First In, First Out (FIFO) principle. The primary operations are enqueue (inserting an element at the end) and dequeue (removing an element from the front).
What is a binary tree?
A binary tree is a tree data structure where each node has at most two children, referred to as the left child and the right child. Binary trees are used in various applications, such as searching and sorting algorithms.
Describe what a hash table is.
A hash table is a data structure that stores key-value pairs. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
Can you explain recursion?
Recursion is a programming technique where a function calls itself in order to solve a problem. It often simplifies the code for problems that can be divided into similar subproblems, such as the computation of factorials or Fibonacci numbers.
What is a pointer and how is it used?
A pointer is a variable that stores the memory address of another variable. Pointers are used for dynamic memory allocation, accessing arrays, and implementing data structures like linked lists and trees.
Explain the concept of a null pointer.
A null pointer is a pointer that does not point to any valid memory location. It is often used to signify that the pointer is not currently pointing to an object.
What are the differences between stack and heap memory?
Stack memory is used for static memory allocation, such as local variables and function calls, and follows the LIFO principle. Heap memory is used for dynamic memory allocation, where variables are allocated and freed in any order.
What is dynamic programming?
Dynamic programming is an optimization technique used to solve complex problems by breaking them down into simpler subproblems. It stores the results of subproblems to avoid redundant computations.
Can you explain what a compiler does?
A compiler is a program that translates source code written in a high-level programming language into machine code, which can be executed by a computer’s CPU. It performs various stages of translation, including lexical analysis, syntax analysis, and code generation.
Describe what an interpreter is.
An interpreter directly executes instructions written in a high-level programming language without converting them into machine code. It reads and executes the code line by line, making it useful for scripting and prototyping.
What is the difference between a compiler and an interpreter?
A compiler translates the entire source code into machine code before execution, while an interpreter translates and executes code line by line. Compilers generally produce faster executable code, while interpreters offer more flexibility and ease of debugging.
Explain the concept of garbage collection.
Garbage collection is the process of automatically reclaiming memory that is no longer in use by the program. It helps prevent memory leaks by identifying and freeing unused objects.
What is a memory leak?
A memory leak occurs when a program fails to release memory that is no longer needed, leading to a gradual decrease in available memory. This can eventually cause the program to crash or slow down.
Can you explain what a segmentation fault is?
A segmentation fault is an error that occurs when a program tries to access a restricted area of memory. It is often caused by dereferencing null or uninitialized pointers or accessing memory outside the bounds of an array.
What are the four principles of OOP (Object-Oriented Programming)?
The four principles of OOP are encapsulation, inheritance, polymorphism, and abstraction. These principles help organize and manage complex software systems by promoting code reuse and modularity.
Describe what inheritance is in OOP.
Inheritance is a mechanism in OOP that allows one class (subclass) to inherit properties and behaviors from another class (superclass). It promotes code reuse and establishes a hierarchical relationship between classes.
Can you explain polymorphism?
Polymorphism is the ability of objects to take on multiple forms. In OOP, it allows methods to be defined in a superclass and overridden in subclasses, enabling different implementations of the same method.
What is encapsulation in OOP?
Encapsulation is the practice of bundling data (attributes) and methods (functions) that operate on the data into a single unit, called a class. It restricts direct access to some of the object’s components, enhancing security and modularity.
Describe abstraction in OOP.
Abstraction is the concept of hiding complex implementation details and exposing only the essential features of an object. It helps manage complexity by allowing the programmer to focus on high-level operations.
What is a constructor in programming?
A constructor is a special method in a class that is automatically called when an object of the class is created. It is used to initialize the object’s attributes and perform any setup required.
Explain what a destructor is.
A destructor is a method in a class that is automatically called when an object of the class is destroyed. It is used to release resources allocated to the object and perform any necessary cleanup.
What is a virtual function?
A virtual function is a function declared in a base class that can be overridden in derived classes. It enables dynamic binding and allows derived classes to provide specific implementations for the function.
Can you describe method overloading?
Method overloading is a feature in programming that allows multiple methods with the same name but different parameter lists to be defined in the same class. It enables the creation of methods that perform similar operations with different inputs.
What is method overriding?
Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. It allows the subclass to customize or extend the behavior of the method.
Explain the concept of a class.
A class is a blueprint for creating objects in OOP. It defines a set of attributes (data) and methods (functions) that describe the behavior and state of objects created from the class.
What is an object in programming?
An object is an instance of a class. It represents a real-world entity with attributes and behaviors defined by the class. Objects are used to model and interact with the world in OOP.
Describe what a singleton class is.
A singleton class is a class that restricts the instantiation of its objects to a single instance. It ensures that only one object of the class exists, providing a global point of access to the instance.
Can you explain what an interface is?
An interface is a contract in programming that defines a set of methods that a class must implement. It allows different classes to adhere to the same protocol, promoting loose coupling and flexibility.
What is an abstract class?
An abstract class is a class that cannot be instantiated and is intended to be subclassed. It may contain abstract methods (methods without implementation) that must be implemented by its subclasses.
Describe what a software design pattern is.
A software design pattern is a general, reusable solution to a common problem in software design. Design patterns provide a standard terminology and are a proven way to address recurring design challenges.
What is the Singleton pattern?
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. It is useful when exactly one object is needed to coordinate actions across a system.
Can you explain the Factory pattern?
The Factory pattern is a creational design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. It promotes loose coupling by delegating the instantiation logic to factory classes.
What is the Observer pattern?
The Observer pattern is a behavioral design pattern where an object (the subject) maintains a list of its dependents (observers) and notifies them of any state changes. It is useful for implementing distributed event-handling systems.
Describe the Model-View-Controller (MVC) pattern.
The MVC pattern is an architectural pattern that separates an application into three main components: the model (data), the view (user interface), and the controller (business logic). It promotes separation of concerns and improves maintainability.
What is the difference between a process and a thread?
A process is an independent program in execution with its own memory space, while a thread is a lightweight subprocess that shares the same memory space with other threads in the same process. Threads allow parallel execution within a process.
Explain multithreading.
Multithreading is a technique where multiple threads are executed concurrently within the same process. It allows for parallelism and efficient use of CPU resources, improving the performance of applications with multiple tasks.
What is a deadlock in programming?
A deadlock occurs when two or more threads or processes are blocked forever, waiting for each other to release resources. It typically happens when threads have circular dependencies on shared resources.
Can you describe what a race condition is?
A race condition occurs when the behavior of a program depends on the relative timing of events, such as the order in which threads execute. It can lead to unpredictable and incorrect results if not properly synchronized.
What is a semaphore?
A semaphore is a synchronization primitive used to control access to a shared resource by multiple threads. It maintains a counter that represents the number of available resources and allows threads to acquire or release resources in a controlled manner.
Explain what a mutex is.
A mutex (mutual exclusion) is a synchronization primitive that ensures only one thread can access a critical section of code at a time. It helps prevent race conditions by providing exclusive access to shared resources.
What is a critical section?
A critical section is a part of a program that accesses shared resources and must not be concurrently executed by more than one thread. Proper synchronization mechanisms, such as mutexes, are used to protect critical sections.
Describe what a lambda function is.
A lambda function is an anonymous function defined using the lambda keyword. It is a small, single-expression function that can be used wherever function objects are required. Lambda functions are often used for short, throwaway functions.
What is the difference between synchronous and asynchronous programming?
Synchronous programming executes tasks sequentially, waiting for each task to complete before moving on to the next one. Asynchronous programming allows tasks to run concurrently, enabling other tasks to proceed without waiting for long-running operations to finish.
Explain what RESTful web services are.
RESTful web services are web services that adhere to the principles of Representational State Transfer (REST). They use standard HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations on resources, which are identified by URLs.
Tips to Answer the Programming Interview Questions
1. Understand the Basics
You can make sure you have a strong grasp of fundamental concepts like data structures, algorithms, and object-oriented programming. A solid foundation will help you tackle a wide range of questions. Delving into the core principles ensures that you can apply them to different scenarios and understand the underlying logic behind the questions. Revisiting your college textbooks or taking online courses can refresh these essential topics.
2. Practice Coding
You should solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal to improve your problem-solving skills and get familiar with different types of questions. Regular practice helps you recognize patterns and common problem types, making it easier to approach new challenges. Additionally, try participating in coding competitions to simulate the time constraints and pressure of an actual interview.
3. Clarify the Question
You can always ask the interviewer for clarification if you don’t understand a question. This shows that you are thorough and want to provide the best possible answer. Misunderstanding a question can lead to incorrect or suboptimal solutions, so it’s better to ask questions and ensure you fully comprehend what is being asked. This also demonstrates your communication skills and attention to detail.
4. Think Aloud
You should explain your thought process while solving a problem. This helps the interviewer understand your approach and reasoning, and it can also give you time to think. Verbalizing your thoughts can highlight your analytical abilities and problem-solving strategy, providing insight into your approach. It also opens the door for feedback or hints from the interviewer, which can guide you towards the right solution.
5. Optimize Your Solution
You can focus on writing clean and efficient code. After solving a problem, you should discuss any potential optimizations and their trade-offs with the interviewer. Optimization involves not just making the code run faster but also using memory efficiently. Discussing different approaches and their complexities (time and space) shows that you are mindful of the best practices and aware of real-world constraints.
6. Use Pseudocode
You can start with pseudocode if you’re unsure about the exact syntax. This helps you organize your thoughts and ensures that you cover the logic before diving into the actual code. Pseudocode allows you to break down the problem into manageable steps and outline the solution structure without worrying about syntax errors. Once the logic is clear, translating it into actual code becomes much easier.
7. Know Your Language
You should be familiar with the syntax, libraries, and common functions of the programming language you’re using. Knowing the standard library can save you time during the interview. Being proficient in your chosen language allows you to write code quickly and efficiently. It also helps you leverage built-in functions and libraries that can simplify complex tasks, showcasing your depth of knowledge and resourcefulness.
8. Test Your Code
You can test your code with different inputs to ensure it handles edge cases and is bug-free. Mention any test cases you would use to validate your solution. Testing demonstrates your ability to write robust, reliable code and shows that you are thorough in your work. Discussing edge cases like empty inputs, large inputs, or special characters also indicates your foresight and attention to detail.
9. Discuss Alternatives
You should discuss alternative approaches and why you chose your specific solution. This shows your understanding of different methods and their pros and cons. Highlighting alternative solutions demonstrates your comprehensive understanding of the problem and your ability to evaluate multiple options. It also shows that you are not fixated on one approach and are open to considering different strategies.
10. Stay Calm
You can remain calm and composed, even if you’re stuck. Take a deep breath, think through the problem logically, and don’t be afraid to ask for hints or move on to another question if needed. Staying calm under pressure shows resilience and poise, qualities that are valuable in any work environment. If you encounter a difficult question, breaking it down into smaller parts or discussing it with the interviewer can often lead to a solution.
Word Of Advice
Programming interviews can be daunting, but with thorough preparation, you can approach them with confidence. By understanding core concepts, practicing coding, and honing your problem-solving skills, you’ll be well-equipped to handle the questions that come your way.
Remember, interviewers value not just the correctness of your answers but also your ability to think critically and communicate effectively. Stay calm, be clear in your explanations, and view each question as an opportunity to showcase your skills.
With the right mindset, you can turn these challenges into stepping stones toward your next career opportunity.
Pingback: 65+ Sales Interview Questions (With Sample Answers)